WSP 1: Test program (2)
public class MyArrayList<E>
{
private int size;
private E[] data;
private int MAXELEMENTS = 100;
public MyArrayList() {
data = (E[])new Object[MAXELEMENTS];
size = 0;
}
public int getMAXELEMENTS(){
return MAXELEMENTS;
}
public boolean checkSpace()
{
if (size+1<MAXELEMENTS)
return true;
else
return false;
}
public void add(int index, E e) {
if (index < 0 || index > size)
throw new IndexOutOfBoundsException
("Index: " + index + ", Size: " + size);
for (int i = size - 1; i >= index; i--)
data[i + 1] = data[i];
data[index] = e;
size++;
}
public boolean contains(Object e) {
for (int i = 0; i < size; i++)
if (e.equals(data[i])) return true;
return false;
}
public E get(int index) {
if (index < 0 || index >= size)
throw new IndexOutOfBoundsException
("Index: " + index + ", Size: " + size);
return data[index];
}
public E remove(int index) {
if (index < 0 || index >= size)
throw new IndexOutOfBoundsException
("Index: " + index + ", Size: " + size);
E e = data[index];
for (int j = index; j < size - 1; j++)
data[j] = data[j + 1];
data[size - 1] = null;
size--;
return e;
}
public void clear()
{
size = 0;
}
public MyArrayList<E> merge(MyArrayList<E> param)
{
int i=0;
int j=0;
int k=0;
MyArrayList<E> returnArray = new MyArrayList();
if (this.getSize() ==0)
return param;
if (param.getSize()==0)
return this;
if ((this.getSize()+ param.getSize()) > MAXELEMENTS)
throw new IndexOutOfBoundsException
("Combined list out of bounds");
while (i<this.getSize() && j<param.getSize())
{
if (((Comparable)data[i]).compareTo(param.data[j]) <0)
{
returnArray.data[k]= this.data[i];
k++;
i++;
}
else
{
returnArray.data[k]=param.data[j];
k++;
j++;
}
}
if (i < this.getSize())
{
for (i=i;i<getSize();i++) //for starts at current position
{
returnArray.data[k]= this.data[i];
k++;
}
}
if (j < param.getSize())
{
for (j=j;j<param.getSize();j++)
{
returnArray.data[k]=param.data[j];
k++;
}
}
returnArray.size = k;
return returnArray;
}
public String toString() {
String result="[";
for (int i = 0; i < size; i++) {
result+= data[i];
if (i < size - 1) result+=", ";
}
return result.toString() + "]";
}
public int getSize()
{
return size;
}
public boolean sortList() {
E hold;
for (int i = 0; i < size-1; i++)
{
for (int j = 0; j<size-1; j++)
{
if(((Comparable)data[j]).compareTo(data[j+1])>0)
{
hold= data[j+1];
data[j+1]=data[j];
data[j]=hold;
}
}
}
return true;
}
}
public class StackAsMyArrayList<E>
{
MyArrayList<E> theStack;
public StackAsMyArrayList()
{
theStack = new MyArrayList<E>();
}
public void push(E newElement) //insert at end of array!
{
if (!theStack.checkSpace())
throw new IndexOutOfBoundsException
("Stack out of bounds");
theStack.add(theStack.getSize(),newElement);
}
public E pop() //remove end of array
{
E temp = null;
boolean isDone = false;
if (theStack.getSize() > 0)
temp=theStack.remove(theStack.getSize()-1);
return temp; // temp will be null in special case of empty list
}
public String toString()
{
return theStack.toString();
}
}
![WSP 1: Test program (1)
Create a test class containing a main() method - Call it Watersort
• The test program first should create single bottle
(StackAsArraylist).
• Create objects of the character glass called red, green and blue.
• Use the following type of static variables:
stooik Cherocter reda new Character
• Put ink in the bottles (Push character objects onto the stack)
• Test the getStockSize() and CheckStackUniform() methods
thoroughly.
WSP 1: Test program (2)
If you succeeded you are now ready to add more bottles
• Carefully think about the game. Can you see that each bottle is
one stack. We need 5 bottles. What type of data structure would
you require when you need S objects of the same class?
WSP 1: Test program (2)
If you succeeded you are now ready to add more bottles
• Carefully think about the game. Can you see that each bottle is
one stack. We need 5 bottles. What type of data structure would
you require when you need 5 objects of the same class?
* You can either use and array or a linked list. In this case the
direct access of an array is much easier to use. So you need an
array of 5 bottles. [(Call the array bottles). We are using the
simple built-in array of Java NOT our own MyArraytist class. [We
want direct access without using accessors and mutators.]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0341fd82-0c93-4c8d-bf8f-3ff093efcbdd%2Fd881a2c8-e14f-47cb-9b04-6c9b062a4093%2Fh3sahff_processed.jpeg&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

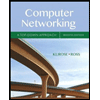
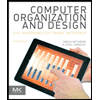
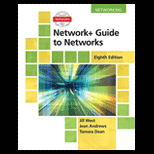
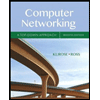
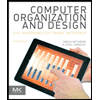
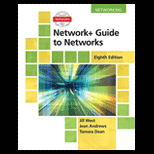
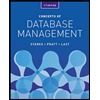
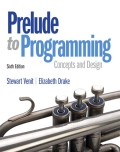
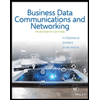