Using c++ BankAccount class Given main(), define the BankAccount class (in files BankAccount.h and BankAccount.cpp) that manages checking and savings accounts. The class has three private data members: customer name (string) savings account balance (double) checking account balance (double) Implement the following constructor and public member functions as listed below: BankAccount(string newName, double amt1, double amt2) - set the customer name to parameter newName, set the checking account balance to parameter amt1 and set the savings account balance to parameter amt2. (amt stands for amount) void SetName(string newName) - set the customer name to parameter newName string GetName() - return the customer name void SetChecking(double amt) - set the checking account balance to parameter amt double GetChecking() - return the checking account balance void SetSavings(double amt) - set the savings account balance to parameter amt double GetSavings() - return the savings account balance void DepositChecking(double amt) - add parameter amt to the checking account balance (only if positive) void DepositSavings(double amt) - add parameter amt to the savings account balance (only if positive) void WithdrawChecking(double amt) - subtract parameter amt from the checking account balance (only if positive) void WithdrawSavings(double amt) - subtract parameter amt from the savings account balance (only if positive) void TransferToSavings(double amt) - subtract parameter amt from the checking account balance and add to the savings account balance (only if positive) #ifndef BANKACCOUNTH #define BANKACCOUNTH #include using namespace std; class BankAccount { public: // TODO: Declare public member functions private: // TODO: Declare private data members };
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Using c++
BankAccount class
Given main(), define the BankAccount class (in files BankAccount.h and BankAccount.cpp) that manages checking and savings accounts. The class has three private data members:
- customer name (string)
- savings account balance (double)
- checking account balance (double)
Implement the following constructor and public member functions as listed below:
- BankAccount(string newName, double amt1, double amt2) - set the customer name to parameter newName, set the checking account balance to parameter amt1 and set the savings account balance to parameter amt2. (amt stands for amount)
- void SetName(string newName) - set the customer name to parameter newName
- string GetName() - return the customer name
- void SetChecking(double amt) - set the checking account balance to parameter amt
- double GetChecking() - return the checking account balance
- void SetSavings(double amt) - set the savings account balance to parameter amt
- double GetSavings() - return the savings account balance
- void DepositChecking(double amt) - add parameter amt to the checking account balance (only if positive)
- void DepositSavings(double amt) - add parameter amt to the savings account balance (only if positive)
- void WithdrawChecking(double amt) - subtract parameter amt from the checking account balance (only if positive)
- void WithdrawSavings(double amt) - subtract parameter amt from the savings account balance (only if positive)
- void TransferToSavings(double amt) - subtract parameter amt from the checking account balance and add to the savings account balance (only if positive)
#ifndef BANKACCOUNTH
#define BANKACCOUNTH
#include <string>
using namespace std;
class BankAccount {
public:
// TODO: Declare public member functions
private:
// TODO: Declare private data members
};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

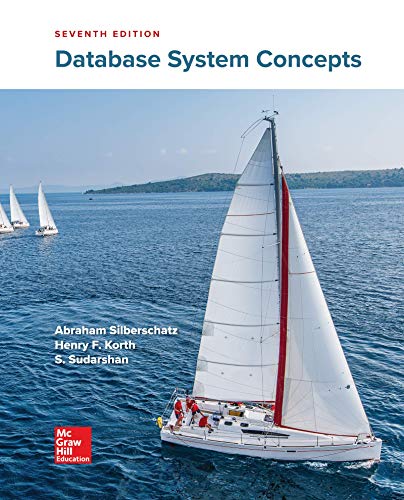
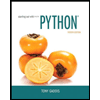
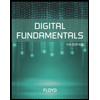
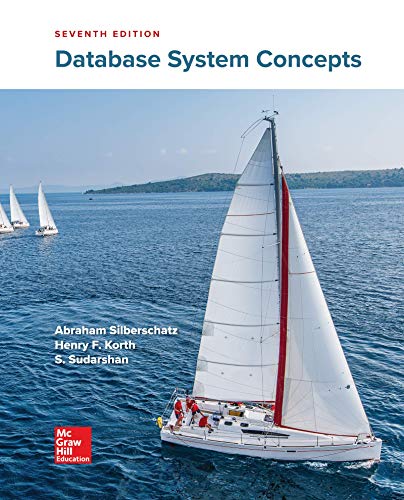
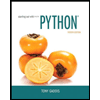
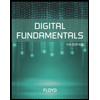
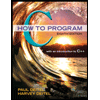
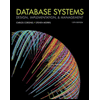
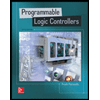