Start by designing a class called Account. This class contains the following private or hidden data attributes: • • acct_num, a unique identifying account number. owner, the name of the owner of the checking account. ⚫ balance, the available balance in the account. The Account class will have the following methods: • An initialization method (_init ) that can be used to create an instance of the Account class. All the data attributes must be specified as arguments. This method prevents initializing the balance to a negative number by assigning zero (0) to the balance attribute and displaying an error message that reports any attempt to initialize the balance data attribute to a negative value. • • • • A deposit method that accepts as an argument the amount of money to be added to the existing balance. If the amount is not a positive number, ignore the transaction and display an error message that reports the attempt. A withdrawal method that attempts to reduce the account balance. This method must not reduce the account balance below zero. If an argument is passed to this method that is not a positive value or would reduce the account balance below zero, ignore the transaction and display an error message instead that reports the attempt. A get_acct_num accessor method that returns the value of the acct num data attribute. A get_owner accessor method that returns the value of the owner data attribute. A get_balance method that returns the value of the balance. Place the class definition for Account in a separate file named "Account.py" and then include an "import Account" statement in your program to gain access to the class definition. Your Python program will read and process two input files and create a single output file as well as displaying limited console output. Your program will read the contents of a file named "accounts.txt" and use the contents to initially create and define several Account objects. The "accounts.txt" text file contains the definition of several accounts. Each record in this file contains the following information in this order: 1. owner's name 2. account number 3. initial balance The fields in this file are comma-separated. That is, each field is separated from the next field by a comma. Example records for this file look like this: Hal Jordan, 12345, 500 Oliver Queen, 23456, 1000 Steve Rogers, 67543,250 Barry Allen, 12507, 450 Bruce Wayne, 78234,2500 Arthur Curry, 87123,560 Diana Prince, 65439, 3250 After creating and initializing instances of the Account class, display a line on the console (screen) using a print statement that reports how many accounts were created. Your program will then download, read, and process a file named "activity.txt". The "activity.txt" text file contains transaction activit that will potentially change the balances of some accounts. The "activity.txt" text file is also a comma delimited file. Individual fields within records are separated from each other by a comma (, ). These are 1 within records are separated from each other by a comma (, ). These are the codes used for the possible transaction activities: "D" Deposit money in the checking account. "W" Withdraw money from the checking account. Records within the "activity.txt" text file contain the following fields: 1. Transaction code ("D" or "w") 2. account number 3. amount of money to be processed. Example records for the "activity.txt" file look like this: D, 12345, 3500 W, 12345, 1001.45 X, 12345, 450 W, 23456, 120 D, 23456, 450 D, 34567,125.50 Only process records in the "activity.txt" file that affect accounts you created earlier (using the "accounts.txt" file) AND contain a valid transaction code ("D" or "w"). Keep track of the total number of activity records processed as well as the number of records that were skipped either because they did not have a valid transaction code, or they dealt with an account that was not created earlier (no matching account number). After processing the transactions in the "activity.txt" file your program will now display two items on the console (screen) using the print statement. You will know and can display the total number of activity records processed as well as the number of activity records skipped. The final step in this programming project is to create an output file that contains an account summary. The account summary will show each account and its balance as well as the grand total of all account balances. This information will be written to an output text file named "Report.txt". The first line of your inventory report will contain a made-up (fictitious) name for the banking institution such as "Albatross Banking, Ltd." Use your imagination and make up your own name for the company. Do not use "Albatross Banking, Ltd." The second line will be blank. The third line in your report is where the heading information begins. Your inventory report should look as much like this as possible: Albatross Banking, Ltd. ACCOUNT Account Owner SUMMARY Balance 12345 Hal Jordan 550.00 23456 Oliver Queen 500.00 67543 Steve Rogers 3,125.00 12507 Barry Allen 78234 Bruce Wayne 87123 65439 450.00 1,300.00 Arthur Curry Diana Prince 556.78 3,250.00 Total 9,731.78 Once the account summary report is complete, display the overall dollar amount of all the account balances to the screen. Summary of expected screen display messages: NN accounts were created. XXX transaction records processed. YY records skipped. Total for all account balances is $DD, DDD.DD Your program will display the number of accounts created in place of NIN The 3
Start by designing a class called Account. This class contains the following private or hidden data attributes: • • acct_num, a unique identifying account number. owner, the name of the owner of the checking account. ⚫ balance, the available balance in the account. The Account class will have the following methods: • An initialization method (_init ) that can be used to create an instance of the Account class. All the data attributes must be specified as arguments. This method prevents initializing the balance to a negative number by assigning zero (0) to the balance attribute and displaying an error message that reports any attempt to initialize the balance data attribute to a negative value. • • • • A deposit method that accepts as an argument the amount of money to be added to the existing balance. If the amount is not a positive number, ignore the transaction and display an error message that reports the attempt. A withdrawal method that attempts to reduce the account balance. This method must not reduce the account balance below zero. If an argument is passed to this method that is not a positive value or would reduce the account balance below zero, ignore the transaction and display an error message instead that reports the attempt. A get_acct_num accessor method that returns the value of the acct num data attribute. A get_owner accessor method that returns the value of the owner data attribute. A get_balance method that returns the value of the balance. Place the class definition for Account in a separate file named "Account.py" and then include an "import Account" statement in your program to gain access to the class definition. Your Python program will read and process two input files and create a single output file as well as displaying limited console output. Your program will read the contents of a file named "accounts.txt" and use the contents to initially create and define several Account objects. The "accounts.txt" text file contains the definition of several accounts. Each record in this file contains the following information in this order: 1. owner's name 2. account number 3. initial balance The fields in this file are comma-separated. That is, each field is separated from the next field by a comma. Example records for this file look like this: Hal Jordan, 12345, 500 Oliver Queen, 23456, 1000 Steve Rogers, 67543,250 Barry Allen, 12507, 450 Bruce Wayne, 78234,2500 Arthur Curry, 87123,560 Diana Prince, 65439, 3250 After creating and initializing instances of the Account class, display a line on the console (screen) using a print statement that reports how many accounts were created. Your program will then download, read, and process a file named "activity.txt". The "activity.txt" text file contains transaction activit that will potentially change the balances of some accounts. The "activity.txt" text file is also a comma delimited file. Individual fields within records are separated from each other by a comma (, ). These are 1 within records are separated from each other by a comma (, ). These are the codes used for the possible transaction activities: "D" Deposit money in the checking account. "W" Withdraw money from the checking account. Records within the "activity.txt" text file contain the following fields: 1. Transaction code ("D" or "w") 2. account number 3. amount of money to be processed. Example records for the "activity.txt" file look like this: D, 12345, 3500 W, 12345, 1001.45 X, 12345, 450 W, 23456, 120 D, 23456, 450 D, 34567,125.50 Only process records in the "activity.txt" file that affect accounts you created earlier (using the "accounts.txt" file) AND contain a valid transaction code ("D" or "w"). Keep track of the total number of activity records processed as well as the number of records that were skipped either because they did not have a valid transaction code, or they dealt with an account that was not created earlier (no matching account number). After processing the transactions in the "activity.txt" file your program will now display two items on the console (screen) using the print statement. You will know and can display the total number of activity records processed as well as the number of activity records skipped. The final step in this programming project is to create an output file that contains an account summary. The account summary will show each account and its balance as well as the grand total of all account balances. This information will be written to an output text file named "Report.txt". The first line of your inventory report will contain a made-up (fictitious) name for the banking institution such as "Albatross Banking, Ltd." Use your imagination and make up your own name for the company. Do not use "Albatross Banking, Ltd." The second line will be blank. The third line in your report is where the heading information begins. Your inventory report should look as much like this as possible: Albatross Banking, Ltd. ACCOUNT Account Owner SUMMARY Balance 12345 Hal Jordan 550.00 23456 Oliver Queen 500.00 67543 Steve Rogers 3,125.00 12507 Barry Allen 78234 Bruce Wayne 87123 65439 450.00 1,300.00 Arthur Curry Diana Prince 556.78 3,250.00 Total 9,731.78 Once the account summary report is complete, display the overall dollar amount of all the account balances to the screen. Summary of expected screen display messages: NN accounts were created. XXX transaction records processed. YY records skipped. Total for all account balances is $DD, DDD.DD Your program will display the number of accounts created in place of NIN The 3
Chapter4: More Object Concepts
Section: Chapter Questions
Problem 11PE
Related questions
Question

Transcribed Image Text:Start by designing a class called Account. This class contains the following
private or hidden data attributes:
•
•
acct_num, a unique identifying account number.
owner,
the name of the owner of the checking account.
⚫ balance, the available balance in the account.
The Account class will have the following methods:
•
An initialization method (_init ) that can be used to create an
instance of the Account class. All the data attributes must be specified
as arguments. This method prevents initializing the balance to a
negative number by assigning zero (0) to the balance attribute and
displaying an error message that reports any attempt to initialize the
balance data attribute to a negative value.
•
•
•
•
A deposit method that accepts as an argument the amount of money
to be added to the existing balance. If the amount is not a positive
number, ignore the transaction and display an error message that
reports the attempt.
A withdrawal method that attempts to reduce the account balance.
This method must not reduce the account balance below zero. If an
argument is passed to this method that is not a positive value or would
reduce the account balance below zero, ignore the transaction and
display an error message instead that reports the attempt.
A get_acct_num accessor method that returns the value of the
acct num data attribute.
A get_owner accessor method that returns the value of the owner
data attribute.
A get_balance method that returns the value of the balance.
Place the class definition for Account in a separate file named "Account.py"
and then include an "import Account" statement in your program to gain
access to the class definition.
Your Python program will read and process two input files and create a
single output file as well as displaying limited console output. Your program
will read the contents of a file named "accounts.txt" and use the contents
to initially create and define several Account objects.
The "accounts.txt" text file contains the definition of several accounts.
Each record in this file contains the following information in this order:
1. owner's name
2. account number
3. initial balance
The fields in this file are comma-separated. That is, each field is separated
from the next field by a comma. Example records for this file look like this:
Hal Jordan, 12345, 500
Oliver Queen, 23456, 1000
Steve Rogers, 67543,250
Barry Allen, 12507, 450
Bruce Wayne, 78234,2500
Arthur Curry, 87123,560
Diana Prince, 65439, 3250
After creating and initializing instances of the Account class, display a line
on the console (screen) using a print statement that reports how many
accounts were created.
Your program will then download, read, and process a file named
"activity.txt". The "activity.txt" text file contains transaction activit
that will potentially change the balances of some accounts. The
"activity.txt" text file is also a comma delimited file. Individual fields
within records are separated from each other by a comma (, ). These are
1

Transcribed Image Text:within records are separated from each other by a comma (, ). These are
the codes used for the possible transaction activities:
"D" Deposit money in the checking account.
"W" Withdraw money from the checking account.
Records within the "activity.txt" text file contain the following fields:
1. Transaction code ("D" or "w")
2. account number
3. amount of money to be processed.
Example records for the "activity.txt" file look like this:
D, 12345, 3500
W, 12345, 1001.45
X, 12345, 450
W, 23456, 120
D, 23456, 450
D, 34567,125.50
Only process records in the "activity.txt" file that affect accounts you
created earlier (using the "accounts.txt" file) AND contain a valid
transaction code ("D" or "w"). Keep track of the total number of activity
records processed as well as the number of records that were skipped either
because they did not have a valid transaction code, or they dealt with an
account that was not created earlier (no matching account number).
After processing the transactions in the "activity.txt" file your program
will now display two items on the console (screen) using the print
statement. You will know and can display the total number of activity
records processed as well as the number of activity records skipped.
The final step in this programming project is to create an output file that
contains an account summary. The account summary will show each account
and its balance as well as the grand total of all account balances. This
information will be written to an output text file named "Report.txt". The
first line of your inventory report will contain a made-up (fictitious) name for
the banking institution such as "Albatross Banking, Ltd." Use your
imagination and make up your own name for the company. Do not use
"Albatross Banking, Ltd." The second line will be blank. The third line in your
report is where the heading information begins. Your inventory report should
look as much like this as possible:
Albatross Banking, Ltd.
ACCOUNT
Account
Owner
SUMMARY
Balance
12345
Hal Jordan
550.00
23456
Oliver Queen
500.00
67543
Steve Rogers
3,125.00
12507 Barry Allen
78234 Bruce Wayne
87123
65439
450.00
1,300.00
Arthur Curry
Diana Prince
556.78
3,250.00
Total
9,731.78
Once the account summary report is complete, display the overall dollar
amount of all the account balances to the screen.
Summary of expected screen display messages:
NN accounts were created.
XXX transaction records processed. YY records skipped.
Total for all account balances is $DD, DDD.DD
Your program will display the number of accounts created in place of NIN
The
3
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
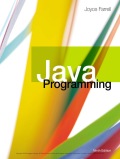
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
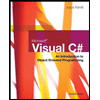
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
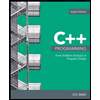
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
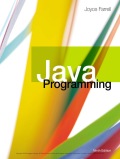
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
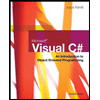
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
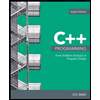
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage