PLEASE DO IN JAVA (for programs like Eclipse) Create a UEmployee class that contains member variables for the university employee name and salary. The UEmployee class should contain member methods for returning the employee name and salary. Create Faculty and Staff classes that inherit the UEmployee class. The Faculty class should include members for storing and returning the department name. The Staff class should include members for storing and returning the job title. User should be able to put in all the information like there name, department and salary. But for department type user should be given options and then told to pick. I have this code, but it does not work for me package UEmployee; import java.util.*; class UEmployee { //delaration of name and salary String empName; double salary; //constructor of UEmployee UEmployee(String empName,double salary) { this.empName=empName; this.salary=salary; } //function to return the employee name String getName() { return empName; } //function to return the salary double getSalary() { return salary; } } class Faculty extends UEmployee { String deptName; //constructor of the Faculty Faculty(String nm,double sal,String dnm) { //passing the name and salary to superclass constructor super(nm,sal); deptName=dnm; } //function to return department name String getDeptName() { return deptName; } } class Staff extends UEmployee { String jobTitle; //constructor of Staff class Staff(String nm,double sal,String title) { //passing the name and salary to superclass constructor super(nm,sal); jobTitle=title; } //function to return job title String getJobTitle() { return jobTitle; } } //test or driver class class Test { public static void main(String args[]) { //accepting the required values from user using Scanner class String nameF,nameS,dname,title; double salF,salS; Scanner sc=new Scanner(System.in); System.out.print(“\nEnter the faculty name: “); nameF=sc.nextLine(); System.out.print(“Enter the salary: “); salF=sc.nextDouble(); System.out.print(“Enter the department name: “); dname=sc.next(); sc.nextLine(); System.out.print(“\nEnter the staff name: “); nameS=sc.nextLine(); System.out.print(“Enter the salary: “); salS=sc.nextDouble(); System.out.print(“Enter the Job title: “); title=sc.next(); //Instantiation of Faculty class Faculty fac=new Faculty(nameF,salF,dname); System.out.println(“\n\nDetails of Faculty:”); System.out.println(“-———————————“); //calling all the methods of Faculty System.out.println(“Name: “+fac.getName()); System.out.println(“Salary: $”+fac.getSalary()); System.out.println(“Departname: “+fac.getDeptName()); //Instantiation of staff class Staff st=new Staff(nameS, salS,title); System.out.println(“\n\nDetails of Staff:”); System.out.println(“-———————————“); //calling all the methods of Staff System.out.println(“Name: “+st.getName()); System.out.println(“Salary: $”+st.getSalary()); System.out.println(“Job Title: “+st.getJobTitle()); System.out.println(“\n”); } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
PLEASE DO IN JAVA (for programs like Eclipse)
Create a UEmployee class that contains member variables for the university employee name and salary.
The UEmployee class should contain member methods for returning the employee name and salary.
Create Faculty and Staff classes that inherit the UEmployee class. The Faculty class should include
members for storing and returning the department name. The Staff class should include members for
storing and returning the job title.
User should be able to put in all the information like there name, department and salary. But for department type user should be given options and then told to pick.
I have this code, but it does not work for me
package UEmployee;
import java.util.*;
class UEmployee
{
//delaration of name and salary
String empName;
double salary;
//constructor of UEmployee
UEmployee(String empName,double salary)
{
this.empName=empName;
this.salary=salary;
}
//function to return the employee name
String getName()
{
return empName;
}
//function to return the salary
double getSalary()
{
return salary;
}
}
class Faculty extends UEmployee
{
String deptName;
//constructor of the Faculty
Faculty(String nm,double sal,String dnm)
{
//passing the name and salary to superclass constructor
super(nm,sal);
deptName=dnm;
}
//function to return department name
String getDeptName()
{
return deptName;
}
}
class Staff extends UEmployee
{
String jobTitle;
//constructor of Staff class
Staff(String nm,double sal,String title)
{
//passing the name and salary to superclass constructor
super(nm,sal);
jobTitle=title;
}
//function to return job title
String getJobTitle()
{
return jobTitle;
}
}
//test or driver class
class Test
{
public static void main(String args[])
{
//accepting the required values from user using Scanner class
String nameF,nameS,dname,title;
double salF,salS;
Scanner sc=new Scanner(System.in);
System.out.print(“\nEnter the faculty name: “);
nameF=sc.nextLine();
System.out.print(“Enter the salary: “);
salF=sc.nextDouble();
System.out.print(“Enter the department name: “);
dname=sc.next();
sc.nextLine();
System.out.print(“\nEnter the staff name: “);
nameS=sc.nextLine();
System.out.print(“Enter the salary: “);
salS=sc.nextDouble();
System.out.print(“Enter the Job title: “);
title=sc.next();
//Instantiation of Faculty class
Faculty fac=new Faculty(nameF,salF,dname);
System.out.println(“\n\nDetails of Faculty:”);
System.out.println(“-———————————“);
//calling all the methods of Faculty
System.out.println(“Name: “+fac.getName());
System.out.println(“Salary: $”+fac.getSalary());
System.out.println(“Departname: “+fac.getDeptName());
//Instantiation of staff class
Staff st=new Staff(nameS, salS,title);
System.out.println(“\n\nDetails of Staff:”);
System.out.println(“-———————————“);
//calling all the methods of Staff
System.out.println(“Name: “+st.getName());
System.out.println(“Salary: $”+st.getSalary());
System.out.println(“Job Title: “+st.getJobTitle());
System.out.println(“\n”);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 6 images

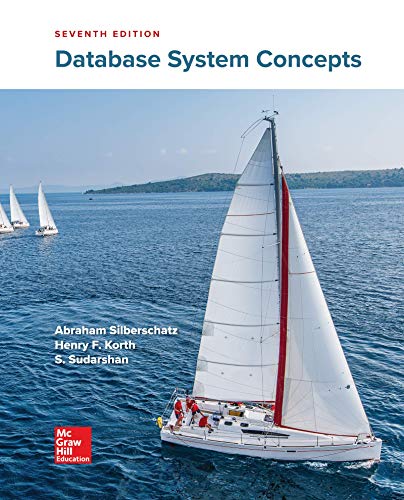
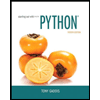
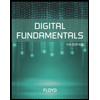
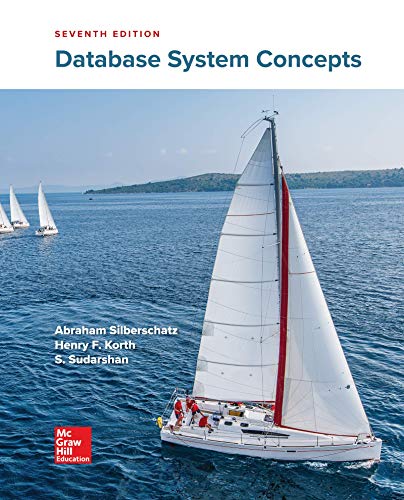
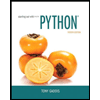
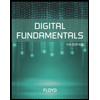
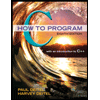
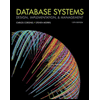
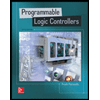