For c++. Need help designing class, no namespsace. FoodWastageRecord NOTE: You need to design this class
For c++. Need help designing class, no namespsace. FoodWastageRecord NOTE: You need to design this class
Chapter8: Arrays
Section: Chapter Questions
Problem 7PE
Related questions
Question
For c++. Need help designing class, no namespsace. FoodWastageRecord
NOTE: You need to design this class.
It represents each food wastage entry recorded by the user through the form on the webpage (frontend).
If you notice the form on the webpage, you’ll see that each FoodWastageRecord will have the following as the data members aka member variables.
Date (as string)
Meal (as string)
Food name (as string)
Quantity in ounces (as double)
Wastage reason (as string)
Disposal mechanism (as string)
Cost (as double)
Each member variable comes with its accessor/mutator functions.
---------------------------------------------------------------------------------
FoodWastageReport
NOTE: You need to design this class.
It represents the report generated on the basis of the records entered by the user. This class will be constructed with all the records entered by the user as a parameter. It will then apply the logic to go over all the records and compute the following:
Names of most commonly wasted foods
Most costly waste producing meals
Total cost of food wasted
Most common reasons of food wastage
Most common mechanisms of food disposal
Suggested strategies to reduce food waste
Your class should have getter functions to retrieve each of the above.
----------------------------------------------
FoodWastageTracker
NOTE: You need to design this class.
It’ll hold all the FoodWastageRecord objects in memory (notice the has-a relationship between the FoodWastageTracker class and FoodWastageRecord class in the diagram above).
It’ll allow the user to
Add a FoodWastageRecord to the list of records in memory. IT SHOULD NOT add duplicate records. Return true if the record was added successfully, else return false.
Delete a FoodWastageRecord from memory. Return true if the record was actually deleted. Return false if the record to be deleted wasn’t found. [NOTE: This is an extra credit requirement.]
Get all FoodWastageRecord objects from memory
Generate and return the FoodWastageReport
All four of these will be the member functions of your class.
======Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
How would you modify these Query files to work with the food_wastage_record.h file
FoodWastageRecord QueryStringToFoodWastageRecord(
const crow::query_string &query_string) {
FoodWastageRecord record;
// TODO 1. Get the date from the query_string using query_string.get("date"),
// and set it in the `record` object using the setter in FoodWastageRecord
// class.
// TODO 2. Get the meal from the query_string using query_string.get("meal"),
// and set it in the `record` object using the setter in FoodWastageRecord
// class.
// TODO 3. Get the food name from the query_string using
// query_string.get("food_name"), and set it in the `record` object using the
// setter in FoodWastageRecord class.
// TODO 4. Get the quantity from the query_string using
// std::stod(query_string.get("qty_in_oz")), and set it in the `record` object
// using the setter in FoodWastageRecord class.
// TODO 5. Get the wastage reason from the query_string using
// query_string.get("wastage_reason"), and set it in the `record` object using
// the setter in FoodWastageRecord class.
// TODO 6. Get the disposal mechanism from the query_string using
// query_string.get("disposal_mechanism"), and set it in the `record` object
// using the setter in FoodWastageRecord class.
// TODO 7. Get the cost from the query_string using
// std::stod(query_string.get("cost")), and set it in the `record` object
// using the setter in FoodWastageRecord class.
return record;
}
crow::json::wvalue FoodWastageTrackerBackend::AddRecord(
const crow::query_string &query_string) {
FoodWastageRecord record = QueryStringToFoodWastageRecord(query_string);
crow::json::wvalue status;
bool add_result = false;
// TODO: Call the member function in the FoodWastageTracker class, on the
// member object that you added in food_wastage_tracker.h, that adds a
// `record` and returns the status of the add operation as a bool. Store the
// returned value in the bool declared above.
status["success"] = add_result;
return status;
}
crow::json::wvalue FoodWastageTrackerBackend::DeleteRecord(
const crow::query_string &query_string) {
FoodWastageRecord record = QueryStringToFoodWastageRecord(query_string);
crow::json::wvalue status;
bool delete_result = false;
// TODO: Call the member function in the FoodWastageTracker class, on the
// member object that you added in food_wastage_tracker.h, that deletes
// `record` and returns the status of the delete operation as a bool. Store
// the returned value in the bool declared above.
status["success"] = delete_result;
return status;
}
===================
food_wastage_record.h file
#include <string>
class FoodWastageRecord {
private:
std::string date;
std::string meal;
std::string foodName;
double quantityInOunces;
std::string wastageReason;
std::string disposalMechanism;
double cost;
public:
// Constructor
FoodWastageRecord(std::string date, std::string meal, std::string foodName,
double quantityInOunces, std::string wastageReason,
std::string disposalMechanism, double cost);
// Accessor and Mutator functions for each member variable
std::string getDate() const;
void setDate(const std::string& date);
std::string getMeal() const;
void setMeal(const std::string& meal);
std::string getFoodName() const;
void setFoodName(const std::string& foodName);
double getQuantityInOunces() const;
void setQuantityInOunces(double quantityInOunces);
std::string getWastageReason() const;
void setWastageReason(const std::string& wastageReason);
std::string getDisposalMechanism() const;
void setDisposalMechanism(const std::string& disposalMechanism);
double getCost() const;
void setCost(double cost);
};
Solution
Follow-up Question
Now that I understand the classes, I must implement them within a backend file. How would I do this in the following?
class FoodWastageTrackerBackend {
public:
FoodWastageTrackerBackend(const std::string &food_wastage_records_filepath)
: food_wastage_records_filepath_{food_wastage_records_filepath} {}
// Reads the food wastage records from the file at
// food_wastage_records_filepath_, and loads those in memory. If the file is
// empty, it is a no-op. Returns true if the file was read successfully, else
// returns false. NOTE: This function should be called right after the object
// instantiation.
//
// Example:
// FoodWastageTrackerBackend backend{"path/to/some_file_name.JSON"};
// backend.LoadRecordsFromJsonFile();
bool LoadRecordsFromJSONFile();
// Writes the food wastage records from the memory to a JSON file at path
// food_wastage_records_filepath_. Returns true if the file was written
// successfully, else returns false.
bool WriteRecordsToJSONFile() const;
// Add the given food wastage record (in the query_string) to the memory.
crow::json::wvalue AddRecord(const crow::query_string &query_string);
// Delete the given food wastage record (in the query_string) from the memory.
crow::json::wvalue DeleteRecord(const crow::query_string &query_string);
// Returns all the food wastage records that are stored in the memory.
crow::json::wvalue GetRecords() const;
// Generate a report based on all the food wastage records in the memory.
crow::json::wvalue GetFoodWastageReport() const;
private:
// File path of the file that stores all the food wastage records in JSON
// format.
const std::string &food_wastage_records_filepath_;
// A top level class object to track the food wastage.
// TODO: Add a FoodWastageTracker object as a member here. Make sure you stick
// to the Google's style guide while naming your variable.
};
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
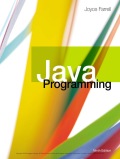
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
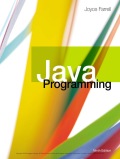
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage