bers also have the ability to be displayed in an original form, decimal form or reduced form. You are going to be completing various object methods to perform the tasks previously list. You are to begin with the Rational Class and RationalTest classes provided. Make all modifications outlined in the point values below. Pay close attention to the names of the attributes, methods and return values. You are given starter files and the test files necessary. Only modify class Rational and keep the function headers provided to you for all of Part 1. You will need to add your own methods for Part 2. Part 1a
You are going to be working with class Rational which defines rational number objects. A rational number is a number that can be written as a fraction. A rational number consists of a numerator and a denominator (which cannot be 0). Rational numbers also have the ability to be displayed in an original form, decimal form or reduced form. You are going to be completing various object methods to perform the tasks previously list.
You are to begin with the Rational Class and RationalTest classes provided. Make all modifications outlined in the point values below. Pay close attention to the names of the attributes, methods and return values. You are given starter files and the test files necessary. Only modify class Rational and keep the function headers provided to you for all of Part 1. You will need to add your own methods for Part 2.
Part 1a
This version requires that you complete the constructor, getOriginal(), and getDecimal() methods of class Rational.
- Constructor: at this stage should have a parameter list that accepts two arguments, a numerator and denominator that are defaulted appropriately. These parameters must initialize attributes called originalNumerator and originalDenominator
- getOriginal(self) should return a string representation of the original fraction. This method will be used in displayData()
- getDecimal(self) should return a string representation of the original fraction as a decimal. This method will be used in displayData().
Part 1b
This version requires that you first modify the constructor, complete method reduce() and getRational(), and lastly modify the displayData method
- Constructor: this needs two new attributes, reducedNumerator and reducedDenominator. You can initialize these to the same values as the origingalNumerator and originalDenominator. The last line of the constructor should be a call to the reduce method that will give them actuall value
- reduce(self) : the reduce method is a non return method. It is to give value to the reducedNumerator and reduceDenominator attributes. Use the getGCF method to help you reduce the original numerator and denominators
- getRational(self) will return a string representation of the rational number in reduced form.
- The new display data method should read:
For Part 2 you are going to need to test your fill with the RationalTestPart2.py file.
You are going to be adding 4 static methods to class rational that will return a rational number that is the sum, difference, product and quotient of two other rational numbers. See the complex number subraction function example in the OOP Part 4 video.
Part 2a Version
This version requires that you write the static multiply and divide methods. These methods will accept two Rational Numbers as parameters and return a new Rational number that is the product or quotient of the two parameters.
The methods must be static method and must be named appropriately
Part 2b Version
The final version requires that you write the static add and subtract methods. These methods will accept two rational numbers as parameters and return a new Rational number that is the sum or difference of the two parameters.
Rational.py
class Rational(object):
"""This creates a rational number (fraction)"""
def __init__(self, numerator=0, denominator=1):
"""Constructor for a rational number with a numerator and denominator"""
pass #remove pass instruction before you begin
def getOriginal(self):
"""returns a string representation of the original rational number"""
pass #remove pass instruction before you begin
def getDecimal(self):
"""returns a string representatin of the rational number in decimal form"""
pass #remove pass instruction before you begin
def reduce(self):
"""reduces the rational number """
pass #remove pass instruction before you begin
def getRational(self):
"""return a string representation of the reduced rational number"""
pass #remove pass instruction before you begin
def displayData(self):
print()
print(self.getOriginal() + " equals "+ self.getDecimal())
print()
def getGCF(self,num1:int, num2:int):
"""returns the greatest common factor of 2 integer values"""
rem = None
gcf = None
while (rem != 0):
rem = num1 % num2
if (rem == 0):
gcf = num2
else:
num1 = num2
num2 = rem
return gcf;
in python please. all the info is here.



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

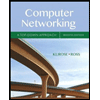
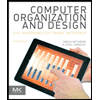
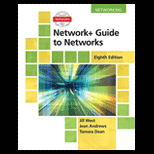
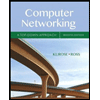
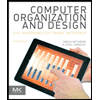
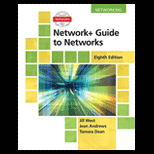
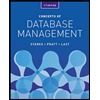
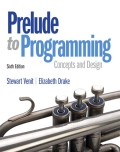
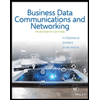